python Selenium 庫的使用技巧
Selenium 是一個用于Web應用程序測試的工具。Selenium測試直接運行在瀏覽器中,就像真正的用戶在操作一樣。支持的瀏覽器包括IE,Mozilla Firefox,Safari,Google Chrome,Opera等。這個工具的主要功能包括:測試與瀏覽器的兼容性——測試你的應用程序看是否能夠很好得工作在不同瀏覽器和操作系統之上。測試系統功能——創建回歸測試檢驗軟件功能和用戶需求。支持自動錄制動作和自動生成 .Net、Java、Perl等不同語言的測試腳本。 -- 百度百科
首先下載驅動文件:https://chromedriver.storage.googleapis.com/index.html?path=2.39/
放入google目錄下
測試代碼,測試是否能讀取到驅動文件。
from selenium import webdriverpath = 'C:/Users/LyShark/AppData/Local/Google/Chrome/Application/chromedriver.exe'driver = webdriver.Chrome(executable_path=path)url = 'https://www.baidu.com'driver.get(url)print(driver.page_source)
簡單的實現瀏覽器測試
# -*- coding:utf-8 -*-from selenium import webdriverWebPath = 'C:/Users/LyShark/AppData/Local/Google/Chrome/Application/chromedriver.exe'driver = webdriver.Chrome(executable_path=WebPath)driver.set_window_size(1000,500)url = 'https://www.baidu.com'driver.get(url)print(driver.find_element_by_id('kw'))
Selenium 自動化測試庫的使用:
<!DOCTYPE html><html lang='en'><head> <meta charset='gbk'> <title>Selenium Test</title></head><body> <div id='aid'> <a rel='external nofollow' name='trnews'>新聞</a> <a rel='external nofollow' name='myblog'>我的博客</a> <a rel='external nofollow' name='mygit'>GitHub</a> </div> <form name='submit_form' action='index.html'> <span class='soutu-btn'></span> <p>用戶: <input name='wd' value='' maxlength='255' autocomplete='off'></p> <p>密碼: <input name='wd' value='' maxlength='255' autocomplete='off'></p> <input type='submit' value='提交' /> </form> <p name='p1' > hello lyshark p1</p> <p name='p2' > hello lyshark p2</p></body></html>
通過簡單的瀏覽文件并實現簡單的定位.
# 驅動下載地址: http://chromedriver.storage.googleapis.com/index.htmlfrom selenium import webdriverWebPath = 'C:/Users/LyShark/AppData/Local/Google/Chrome/Application/chromedriver.exe'driver = webdriver.Chrome(executable_path=WebPath)driver.set_window_size(1024,768)# 常用的定位變量參數如下所示.driver.get('http://lyshark.com')print('當前URL: {}'.format(driver.current_url))print('當前標題: {}'.format(driver.title))print('網頁代碼: {}'.format(driver.page_source))# 基本的 find_element 標簽查找定位方式print(driver.find_element_by_id('user')) # 通過ID來查找元素print(driver.find_element_by_name('p1').text) # 通過name屬性來定位print(driver.find_element_by_class_name('s_ipt')) # 通過類名來定位# 通過xpath定位,xpath定位有N種寫法,這里列幾個常用寫法print(driver.find_element_by_xpath('//form[@class=’fms’]//input[@id=’user’]'))print(driver.find_element_by_xpath('//p[@name=’p1’]'))print(driver.find_element_by_xpath('//html/body/form/p/input'))print(driver.find_elements_by_css_selector('.fms #user'))# 定位a標簽中的關鍵字.print(driver.find_element_by_link_text('新聞'))print(driver.find_element_by_partial_link_text('我'))
通過xpath定位標簽并自動輸入內容,發送登錄請求到后端,寫法如下.
from selenium import webdriverWebPath = 'C:/Users/LyShark/AppData/Local/Google/Chrome/Application/chromedriver.exe'driver = webdriver.Chrome(executable_path=WebPath)driver.set_window_size(1024,768)driver.get('http://lyshark.com')# 通過xpath語法定位到用戶名的標簽上并且自動輸入lyshark這個用戶名driver.find_element_by_xpath('//form[@class=’fms’]/p//input[@id=’user’]').send_keys('lyshark')# 通過xpath語法定位到密碼的標簽上清空默認值,然后輸入123123密碼driver.find_element_by_xpath('//form[@class=’fms’]/p//input[@id=’pass’]').clear()driver.find_element_by_xpath('//form[@class=’fms’]/p//input[@id=’pass’]').send_keys('123123')# 提交這個請求,默認有兩種提交方式一種是 click() 一種是submit()driver.find_element_by_xpath('//form[@class=’fms’]/input[@type=’submit’]').click()
通過鍵盤鼠標類庫記錄并可回放
from selenium import webdriverfrom selenium.webdriver import ActionChainsfrom selenium.webdriver.common.keys import KeysWebPath = 'C:/Users/LyShark/AppData/Local/Google/Chrome/Application/chromedriver.exe'driver = webdriver.Chrome(executable_path=WebPath)driver.set_window_size(1024,768)driver.get('https://www.baidu.com')# ------------------------------------------------------------------------# ActionChains 類提供了鼠標操作的常用方法,鼠標事件的常用函數說明# perform(): 鼠標懸浮于標簽# context_click(): 右擊# double_click(): 雙擊# drag_and_drop(): 拖動# move_to_element():鼠標懸停# 定位到要懸停的元素above = driver.find_element_by_link_text('更多產品')# 對定位到的元素執行鼠標懸停操作ActionChains(driver).move_to_element(above).perform()# ------------------------------------------------------------------------# webdriver.common.keys 類提供了鍵盤事件的操作,以下為常用的鍵盤操作:# send_keys(Keys.BACK_SPACE) 刪除鍵(BackSpace)# send_keys(Keys.SPACE) 空格鍵(Space)# send_keys(Keys.TAB) 制表鍵(Tab)# send_keys(Keys.ESCAPE) 回退鍵(Esc)# send_keys(Keys.ENTER) 回車鍵(Enter)# send_keys(Keys.CONTROL,’a’) 全選(Ctrl+A)# send_keys(Keys.CONTROL,’c’) 復制(Ctrl+C)# send_keys(Keys.CONTROL,’x’) 剪切(Ctrl+X)# send_keys(Keys.CONTROL,’v’) 粘貼(Ctrl+V)# send_keys(Keys.F1) 鍵盤 F1# 輸入框輸入內容driver.find_element_by_id('kw').send_keys('seleniumm')# 刪除多輸入的一個 mdriver.find_element_by_id('kw').send_keys(Keys.BACK_SPACE)# 輸入空格鍵+從入門到入土driver.find_element_by_id('kw').send_keys(Keys.SPACE)driver.find_element_by_id('kw').send_keys('從入門到入土')# ctrl+a 全選輸入框內容driver.find_element_by_id('kw').send_keys(Keys.CONTROL, ’a’)# ctrl+x 剪切輸入框內容driver.find_element_by_id('kw').send_keys(Keys.CONTROL, ’x’)# ctrl+v 粘貼內容到輸入框driver.find_element_by_id('kw').send_keys(Keys.CONTROL, ’v’)# 通過回車鍵來代替單擊操作driver.find_element_by_id('su').send_keys(Keys.ENTER)
簡單的點擊事件
# -*- coding:utf-8 -*-from selenium import webdriverimport timeWebPath = 'C:/Users/LyShark/AppData/Local/Google/Chrome/Application/chromedriver.exe'driver = webdriver.Chrome(executable_path=WebPath)driver.set_window_size(1024,768)driver.get('https://www.baidu.com')driver.find_element_by_id('kw').send_keys('lyshark') # 發送給id=kw的編輯框,搜索關鍵字 lysharkdriver.find_element_by_id('su').click()# 點擊搜索按鈕,百度一下的ID是sutime.sleep(1)# xpath 語法 尋找 div id是1里面的 a標簽取出標簽中的 contains text()driver.find_element_by_xpath('//div[@id=’1’]//a[contains(text(),’-’)]').click()time.sleep(1)handle = driver.current_window_handle # 獲取當前窗口句柄handle_all = driver.window_handles # 獲取當前所有開啟窗口的句柄print(handle_all)driver.switch_to.window(handle_all[0]) # 切換到第一個窗口中time.sleep(1)driver.find_element_by_id('kw').clear() # 接著清空搜索框中的內容
百度自動收集
from selenium import webdriverfrom bs4 import BeautifulSoupfrom queue import Queueimport requests,os,re,lxml# driver: http://chromedriver.storage.googleapis.com/index.html?path=79.0.3945.36/head = {'User-Agent':'Mozilla/5.0 (iPhone; U; CPU like Mac OS X) AppleWebKit/420.1 (KHTML, like Gecko) Version/3.0 Mobile/4A93 Safari/419.3'}WebPath = 'C:/Users/LyShark/AppData/Local/Google/Chrome/Application/chromedriver.exe'driver = webdriver.Chrome(executable_path=WebPath)queue = Queue()for item in range(0,1000,10):queue.put(’https://www.baidu.com/s?wd={}&pn={}’.format('lyshark',str(item)))for item in queue.queue:driver.get(item)ret = str(driver.page_source)try:soup = BeautifulSoup(ret,’lxml’)urls = soup.find_all(name=’a’,attrs={’data-click’:re.compile((’.’)),’class’:None})for item in urls: get_url = requests.get(url=item[’href’],headers=head,timeout=5) if get_url.status_code == 200: print(get_url.url)except Exception:pass
頁面等待
from selenium import webdriverdriver=webdriver.Chrome()driver.get(’https://www.taobao.com/’)wait=WebDriverWait(driver,3) #設置監聽driver等待時間3秒input=wait.until(EC.presence_of_element_located((By.ID,’q’))) #設置等待條件為id為q的元素加載完成button=wait.until(EC.element_to_be_clickable((By.CSS_SELECTOR,’.btn-search’))) #設置等待條件為class名為btn-search的元素加載完成print(input,button)driver = webdriver.Firefox()driver.implicitly_wait(10) #隱式等待設置為10等待時間driver.get('http://somedomain/url_that_delays_loading')myDynamicElement = driver.find_element_by_id('myDynamicElement')
鍵盤操作
element=driver.find_element_by_id(’search’) #獲取輸入框element.send_keys(’selenium’) #搜索selenium包element.send_keys(Keys.ENTER) #按回車鍵element_a=driver.find_element_by_link_text(’selenium’) #定位selenium包鏈接ActionChains(driver).move_to_element(element_a).click(element_a).perform() #按左鍵點擊鏈接執行element_down=driver.find_element_by_link_text(’Download files’) #定位下載鏈接ActionChains(driver).move_to_element(element_down).click(element_down).perform() #按左鍵點擊鏈接element_selenium=driver.find_element_by_link_text(’selenium-3.13.0.tar.gz’) #定位元素selenium下載包鏈接data=element_selenium.get_attribute(’href’) #獲取鏈接地址with open(’selenium-3.13.0.tar.gz’,’wb’) as f: source=requests.get(data).content #請求下載鏈接地址獲取二進制包數據 f.write(source) #寫入數據 f.close() driver.quit()menu = driver.find_element_by_css_selector('.nav') #獲取element對象hidden_submenu = driver.find_element_by_css_selector('.nav #submenu1') #獲取點擊對象#創建鼠標對象actions = ActionChains(driver)#移動鼠標到對象actions.move_to_element(menu)#點擊對象actions.click(hidden_submenu)#執行操作actions.perform()
文章作者:lyshark文章出處:https://www.cnblogs.com/lyshark
以上就是python Selenium 庫的使用技巧的詳細內容,更多關于python Selenium 庫的資料請關注好吧啦網其它相關文章!
相關文章:
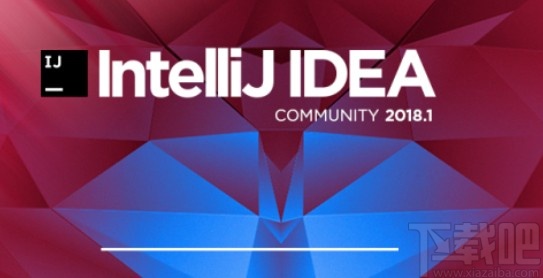