如何在vue中使用video.js播放m3u8格式的視頻
@[toc] 注意:
'vue': '^2.6.11', 'video.js': '^7.10.2', 'videojs-contrib-hls': '^5.15.0', 'mux.js': '^5.7.0'一、安裝
yarn add video.jsyarn add videojs-contrib-hls // 這是播放hls流需要的插件yarn add mux.js // 在vue項(xiàng)目中,若不安裝它可能報(bào)錯(cuò)二、引入videojs
1.在src文件夾下新建 plugins文件夾,并新建video.js文件;
video.js文件的內(nèi)容如下:
import 'video.js/dist/video-js.css'; // 引入video.js的cssimport hls from 'videojs-contrib-hls'; // 播放hls流需要的插件import Vue from 'vue';Vue.use(hls);
2.在main.js中引入剛剛的video.js文件
import './plugins/video.js'; // 引入剛剛定義的video.js文件三、在組件中測(cè)試并使用1. 實(shí)現(xiàn)基本的自動(dòng)播放
Test.vue文件
<template> <div class='test-videojs'> <video muted></video> </div></template><script>import Videojs from 'video.js'; // 引入Videojs export default { data() { return { // https://test-streams.mux.dev/x36xhzz/x36xhzz.m3u8 是一段視頻,可將cctv1 (是live現(xiàn)場(chǎng)直播,不能快退)的替換為它,看到快進(jìn)快退的效果 nowPlayVideoUrl: 'http://ivi.bupt.edu.cn/hls/cctv1hd.m3u8' }; }, mounted() { this.initVideo(this.nowPlayVideoUrl); }, methods: { initVideo(nowPlayVideoUrl) { // 這些options屬性也可直接設(shè)置在video標(biāo)簽上,見(jiàn) muted let options = { autoplay: true, // 設(shè)置自動(dòng)播放 controls: true, // 顯示播放的控件 sources: [ // 注意,如果是以option方式設(shè)置的src,是不能實(shí)現(xiàn) 換臺(tái)的 (即使監(jiān)聽(tīng)了nowPlayVideoUrl也沒(méi)實(shí)現(xiàn)) { src: nowPlayVideoUrl, type: 'application/x-mpegURL' // 告訴videojs,這是一個(gè)hls流 } ] }; // videojs的第一個(gè)參數(shù)表示的是,文檔中video的id const myPlyer = Videojs('videoPlayer', options, function onPlayerReady() { console.log('onPlayerReady 中的this指的是:', this); // 這里的this是指Player,是由Videojs創(chuàng)建出來(lái)的實(shí)例 console.log(myPlyer === this); // 這里返回的是true }); } }};</script><style lang='scss'>#videoPlayer { width: 500px; height: 300px; margin: 50px auto;}</style>
視頻播放效果如圖:
打印結(jié)果如圖:
Test.vue文件
<template> <div class='test-videojs'> <video class='video-js'></video> <el-button type='danger' @click='changeSource'>切換到CCTV3</el-button> </div></template><script>import Videojs from 'video.js'; // 引入Videojsexport default { data() { return { nowPlayVideoUrl: 'http://ivi.bupt.edu.cn/hls/cctv1hd.m3u8', options: { autoplay: true, // 設(shè)置自動(dòng)播放 muted: true, // 設(shè)置了它為true,才可實(shí)現(xiàn)自動(dòng)播放,同時(shí)視頻也被靜音 (Chrome66及以上版本,禁止音視頻的自動(dòng)播放) preload: 'auto', // 預(yù)加載 controls: true // 顯示播放的控件 }, player:null }; }, mounted() { this.getVideo(this.nowPlayVideoUrl); }, methods: { getVideo(nowPlayVideoUrl) { this.player = Videojs('videoPlayer', this.options); //關(guān)鍵代碼, 動(dòng)態(tài)設(shè)置src,才可實(shí)現(xiàn)換臺(tái)操作 this.player.src([ { src: nowPlayVideoUrl, type: 'application/x-mpegURL' // 告訴videojs,這是一個(gè)hls流 } ]); }, changeSource() { this.nowPlayVideoUrl = 'http://ivi.bupt.edu.cn/hls/cctv3hd.m3u8'; console.log(this.nowPlayVideoUrl, '改變了'); } }, watch: { nowPlayVideoUrl(newVal, old) { this.getVideo(newVal); } }, beforeDestroy() { if (this.player) { this.player.dispose(); // Removing Players,該方法會(huì)重置videojs的內(nèi)部狀態(tài)并移除dom } }};</script><style lang='scss'>#videoPlayer { width: 500px; height: 300px; margin: 50px auto;}</style>
視頻切換效果如圖:
需用muted屬性解決
報(bào)錯(cuò)信息:DOMException: play() failedbecause the user didn’t interact with the document first.(用戶還沒(méi)有交互,不能調(diào)用play)
解決辦法:設(shè)置muted屬性為true
<video muted></video>
關(guān)于muted屬性:
muted 屬性,設(shè)置或返回音頻是否應(yīng)該被靜音(關(guān)閉聲音);屬性的值是true和false; muted='false' 表示視頻不用靜音(視頻播放便有聲音),但設(shè)置 muted='fasle' 的情況下,視頻無(wú)法實(shí)現(xiàn)自動(dòng)播放。 video 標(biāo)簽中 muted 的作用: 允許視頻自動(dòng)播放;(Chrome66版本開(kāi)始,禁止視頻和音頻的自動(dòng)播放) 2. 換臺(tái)的時(shí)候,url已經(jīng)成功更改,但視頻還是之前的需得動(dòng)態(tài)設(shè)置src才能實(shí)現(xiàn)
// 動(dòng)態(tài)設(shè)置srcthis.player.src([ { src: nowPlayVideoUrl, type: 'application/x-mpegURL' // 告訴videojs,這是一個(gè)hls流 } ]);3. 找不到mux.js模塊
報(bào)錯(cuò)信息:* mux.js/lib/tools/parse-sidx in ./node_modules/video.js/dist/video.es.js To install it, you can run: npm install --save mux.js/lib/tools/parse-sidx
解決辦法:安裝mux.js
yarn add mux.js五、 播放rtmp流
播放rtmp流的操作與播放hls流的操作幾乎相同,不同在于:
import 'videojs-flash'; // 播放rtmp流需要的插件type: ’rtmp/flv’, // 這個(gè)type值必寫(xiě), 告訴videojs這是一個(gè)rtmp流視頻
以上就是如何在vue中使用video.js 播放m3u8格式的視頻的詳細(xì)內(nèi)容,更多關(guān)于vue 使用videojs 播放m3u8格式的視頻的資料請(qǐng)關(guān)注好吧啦網(wǎng)其它相關(guān)文章!
相關(guān)文章:
1. ASP.Net Core對(duì)USB攝像頭進(jìn)行截圖2. JSP頁(yè)面的靜態(tài)包含和動(dòng)態(tài)包含使用方法3. 通過(guò)Ajax方式綁定select選項(xiàng)數(shù)據(jù)的實(shí)例4. ajax動(dòng)態(tài)加載json數(shù)據(jù)并詳細(xì)解析5. ASP.NET MVC使用Boostrap實(shí)現(xiàn)產(chǎn)品展示、查詢、排序、分頁(yè)6. 通過(guò)CSS數(shù)學(xué)函數(shù)實(shí)現(xiàn)動(dòng)畫(huà)特效7. ASP.Net Core(C#)創(chuàng)建Web站點(diǎn)的實(shí)現(xiàn)8. ASP.NET MVC實(shí)現(xiàn)橫向展示購(gòu)物車(chē)9. .net如何優(yōu)雅的使用EFCore實(shí)例詳解10. 一文透徹詳解.NET框架類(lèi)型系統(tǒng)設(shè)計(jì)要點(diǎn)
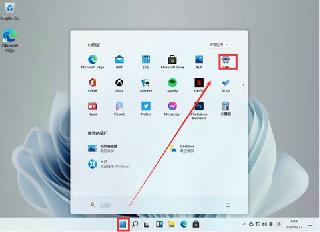