SpringBoot實(shí)現(xiàn)線程池
現(xiàn)在由于系統(tǒng)越來越復(fù)雜,導(dǎo)致很多接口速度變慢,這時(shí)候就會想到可以利用線程池來處理一些耗時(shí)并不影響系統(tǒng)的操作。
新建Spring Boot項(xiàng)目1. ExecutorConfig.xml新建線程池配置文件。
@Configuration@EnableAsyncpublic class ExecutorConfig { private static final Logger logger = LoggerFactory.getLogger(ExecutorConfig.class); @Value('${async.executor.thread.core_pool_size}') private int corePoolSize; @Value('${async.executor.thread.max_pool_size}') private int maxPoolSize; @Value('${async.executor.thread.queue_capacity}') private int queueCapacity; @Value('${async.executor.thread.name.prefix}') private String namePrefix; @Bean(name = 'asyncServiceExecutor') public Executor asyncServiceExecutor() {logger.info('start asyncServiceExecutor');ThreadPoolTaskExecutor executor = new ThreadPoolTaskExecutor();//配置核心線程數(shù)executor.setCorePoolSize(corePoolSize);//配置最大線程數(shù)executor.setMaxPoolSize(maxPoolSize);//配置隊(duì)列大小executor.setQueueCapacity(queueCapacity);//配置線程池中的線程的名稱前綴executor.setThreadNamePrefix(namePrefix);// rejection-policy:當(dāng)pool已經(jīng)達(dá)到max size的時(shí)候,如何處理新任務(wù)// CALLER_RUNS:不在新線程中執(zhí)行任務(wù),而是有調(diào)用者所在的線程來執(zhí)行executor.setRejectedExecutionHandler(new ThreadPoolExecutor.CallerRunsPolicy());//執(zhí)行初始化executor.initialize();return executor; }}2. application.yml
@Value配置在application.yml,可以參考配置
# 異步線程配置async: executor: thread: # 配置核心線程數(shù) core_pool_size: 10 # 配置最大線程數(shù) max_pool_size: 20 # 配置隊(duì)列大小 queue_capacity: 99999 # 配置線程池中的線程的名稱前綴 name:prefix: async-service-3. AsyncService.java
創(chuàng)建一個(gè) Service 接口,是異步線程的接口,將方法寫入其實(shí)現(xiàn)類即可
public interface AsyncService { /** * 執(zhí)行異步任務(wù)的方法,參數(shù)自己可以添加 */ void executeAsync();}4. AsyncServiceImpl.java
實(shí)現(xiàn)類,用來寫業(yè)務(wù)邏輯
@Servicepublic class AsyncServiceImpl implements AsyncService { private static final Logger logger = LoggerFactory.getLogger(AsyncServiceImpl.class); @Override @Async('asyncServiceExecutor') public void executeAsync() {logger.info('start executeAsync');System.out.println('異步線程執(zhí)行開始了');System.out.println('可以將耗時(shí)的操作放到這里執(zhí)行了');logger.info('end executeAsync'); }}
++將 Service 層的服務(wù)異步化,在executeAsync()方法上增加注解@Async('asyncServiceExecutor'),asyncServiceExecutor方法是前面ExecutorConfig.java中的方法名,表明executeAsync方法進(jìn)入的線程池是asyncServiceExecutor方法創(chuàng)建的。++
5. AsyncController.java在控制器里面注入AsyncService,調(diào)用其中的方法即可
@Autowiredprivate AsyncService asyncService;@GetMapping('/async')public void async(){ asyncService.executeAsync();}6. 用Postman進(jìn)行測試
打印log入下
2021-06-16 22:15:47.655 INFO 10516 --- [async-service-5] c.u.d.e.executor.impl.AsyncServiceImpl : start executeAsync異步線程執(zhí)行開始了可以將耗時(shí)的操作放到這里執(zhí)行了2021-06-16 22:15:47.655 INFO 10516 --- [async-service-5] c.u.d.e.executor.impl.AsyncServiceImpl : end executeAsync2021-06-16 22:15:47.770 INFO 10516 --- [async-service-1] c.u.d.e.executor.impl.AsyncServiceImpl : start executeAsync異步線程執(zhí)行開始了可以將耗時(shí)的操作放到這里執(zhí)行了2021-06-16 22:15:47.770 INFO 10516 --- [async-service-1] c.u.d.e.executor.impl.AsyncServiceImpl : end executeAsync2021-06-16 22:15:47.816 INFO 10516 --- [async-service-2] c.u.d.e.executor.impl.AsyncServiceImpl : start executeAsync異步線程執(zhí)行開始了可以將耗時(shí)的操作放到這里執(zhí)行了2021-06-16 22:15:47.816 INFO 10516 --- [async-service-2] c.u.d.e.executor.impl.AsyncServiceImpl : end executeAsync2021-06-16 22:15:48.833 INFO 10516 --- [async-service-3] c.u.d.e.executor.impl.AsyncServiceImpl : start executeAsync異步線程執(zhí)行開始了可以將耗時(shí)的操作放到這里執(zhí)行了2021-06-16 22:15:48.834 INFO 10516 --- [async-service-3] c.u.d.e.executor.impl.AsyncServiceImpl : end executeAsync2021-06-16 22:15:48.986 INFO 10516 --- [async-service-4] c.u.d.e.executor.impl.AsyncServiceImpl : start executeAsync異步線程執(zhí)行開始了可以將耗時(shí)的操作放到這里執(zhí)行了2021-06-16 22:15:48.987 INFO 10516 --- [async-service-4] c.u.d.e.executor.impl.AsyncServiceImpl : end executeAsync至此簡單的線程池已經(jīng)實(shí)現(xiàn)了。
5. 將當(dāng)前線程池的運(yùn)行狀況打印出來5.1 VisiableThreadPoolTaskExecutor.java
public class VisiableThreadPoolTaskExecutor extends ThreadPoolTaskExecutor { private static final Logger logger = LoggerFactory.getLogger(VisiableThreadPoolTaskExecutor.class); private void showThreadPoolInfo(String prefix) {ThreadPoolExecutor threadPoolExecutor = getThreadPoolExecutor();if (null == threadPoolExecutor) { return;}logger.info('{}, {},taskCount [{}], completedTaskCount [{}], activeCount [{}], queueSize [{}]',this.getThreadNamePrefix(),prefix,threadPoolExecutor.getTaskCount(),threadPoolExecutor.getCompletedTaskCount(),threadPoolExecutor.getActiveCount(),threadPoolExecutor.getQueue().size()); } @Override public void execute(Runnable task) {showThreadPoolInfo('1. do execute');super.execute(task); } @Override public void execute(Runnable task, long startTimeout) {showThreadPoolInfo('2. do execute');super.execute(task, startTimeout); } @Override public Future<?> submit(Runnable task) {showThreadPoolInfo('1. do submit');return super.submit(task); } @Override public <T> Future<T> submit(Callable<T> task) {showThreadPoolInfo('2. do submit');return super.submit(task); } @Override public ListenableFuture<?> submitListenable(Runnable task) {showThreadPoolInfo('1. do submitListenable');return super.submitListenable(task); } @Override public <T> ListenableFuture<T> submitListenable(Callable<T> task) {showThreadPoolInfo('2. do submitListenable');return super.submitListenable(task); }}
5.2 修改asyncServiceExecutor.java
修改ExecutorConfig.java的asyncServiceExecutor方法,將ThreadPoolTaskExecutor executor = new ThreadPoolTaskExecutor()改為ThreadPoolTaskExecutor executor = new VisiableThreadPoolTaskExecutor()
@Bean(name = 'asyncServiceExecutor') public Executor asyncServiceExecutor() {logger.info('start asyncServiceExecutor');//在這里修改ThreadPoolTaskExecutor executor = new VisiableThreadPoolTaskExecutor();//配置核心線程數(shù)executor.setCorePoolSize(corePoolSize);//配置最大線程數(shù)executor.setMaxPoolSize(maxPoolSize);//配置隊(duì)列大小executor.setQueueCapacity(queueCapacity);//配置線程池中的線程的名稱前綴executor.setThreadNamePrefix(namePrefix);// rejection-policy:當(dāng)pool已經(jīng)達(dá)到max size的時(shí)候,如何處理新任務(wù)// CALLER_RUNS:不在新線程中執(zhí)行任務(wù),而是有調(diào)用者所在的線程來執(zhí)行executor.setRejectedExecutionHandler(new ThreadPoolExecutor.CallerRunsPolicy());//執(zhí)行初始化executor.initialize();return executor; }
5.3 使用Postman進(jìn)行測試
2021-06-16 22:23:30.951 INFO 14088 --- [nio-8087-exec-2] u.d.e.e.i.VisiableThreadPoolTaskExecutor : async-service-, 2. do submit,taskCount [0], completedTaskCount [0], activeCount [0], queueSize [0]2021-06-16 22:23:30.952 INFO 14088 --- [async-service-1] c.u.d.e.executor.impl.AsyncServiceImpl : start executeAsync異步線程執(zhí)行開始了可以將耗時(shí)的操作放到這里執(zhí)行了2021-06-16 22:23:30.953 INFO 14088 --- [async-service-1] c.u.d.e.executor.impl.AsyncServiceImpl : end executeAsync2021-06-16 22:23:31.351 INFO 14088 --- [nio-8087-exec-3] u.d.e.e.i.VisiableThreadPoolTaskExecutor : async-service-, 2. do submit,taskCount [1], completedTaskCount [1], activeCount [0], queueSize [0]2021-06-16 22:23:31.353 INFO 14088 --- [async-service-2] c.u.d.e.executor.impl.AsyncServiceImpl : start executeAsync異步線程執(zhí)行開始了可以將耗時(shí)的操作放到這里執(zhí)行了2021-06-16 22:23:31.353 INFO 14088 --- [async-service-2] c.u.d.e.executor.impl.AsyncServiceImpl : end executeAsync2021-06-16 22:23:31.927 INFO 14088 --- [nio-8087-exec-5] u.d.e.e.i.VisiableThreadPoolTaskExecutor : async-service-, 2. do submit,taskCount [2], completedTaskCount [2], activeCount [0], queueSize [0]2021-06-16 22:23:31.929 INFO 14088 --- [async-service-3] c.u.d.e.executor.impl.AsyncServiceImpl : start executeAsync異步線程執(zhí)行開始了可以將耗時(shí)的操作放到這里執(zhí)行了2021-06-16 22:23:31.930 INFO 14088 --- [async-service-3] c.u.d.e.executor.impl.AsyncServiceImpl : end executeAsync2021-06-16 22:23:32.496 INFO 14088 --- [nio-8087-exec-7] u.d.e.e.i.VisiableThreadPoolTaskExecutor : async-service-, 2. do submit,taskCount [3], completedTaskCount [3], activeCount [0], queueSize [0]2021-06-16 22:23:32.498 INFO 14088 --- [async-service-4] c.u.d.e.executor.impl.AsyncServiceImpl : start executeAsync異步線程執(zhí)行開始了可以將耗時(shí)的操作放到這里執(zhí)行了2021-06-16 22:23:32.499 INFO 14088 --- [async-service-4] c.u.d.e.executor.impl.AsyncServiceImpl : end executeAsync
可以看到上面async-service-, 2. do submit,taskCount [3], completedTaskCount [3], activeCount [0], queueSize [0]關(guān)于線程的信息都打印出來了。
到此這篇關(guān)于SpringBoot實(shí)現(xiàn)線程池的文章就介紹到這了,更多相關(guān)SpringBoot 線程池內(nèi)容請搜索好吧啦網(wǎng)以前的文章或繼續(xù)瀏覽下面的相關(guān)文章希望大家以后多多支持好吧啦網(wǎng)!
相關(guān)文章:
1. XML入門精解之結(jié)構(gòu)與語法2. html清除浮動的6種方法示例3. CSS3實(shí)現(xiàn)動態(tài)翻牌效果 仿百度貼吧3D翻牌一次動畫特效4. 原生js XMLhttprequest請求onreadystatechange執(zhí)行兩次的解決5. 刪除docker里建立容器的操作方法6. msxml3.dll 錯(cuò)誤 800c0019 系統(tǒng)錯(cuò)誤:-2146697191解決方法7. asp在iis7報(bào)錯(cuò)行號不準(zhǔn)問題的解決方法8. asp批量添加修改刪除操作示例代碼9. 三個(gè)不常見的 HTML5 實(shí)用新特性簡介10. ASP中解決“對象關(guān)閉時(shí),不允許操作。”的詭異問題……
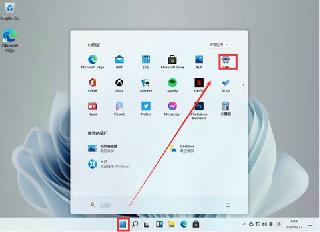