Java 使用Thumbnails對大圖片壓縮
引言
在最近的項目開發中,經常會使用到圖片上傳,但是過大的圖片在查看的時候會影響打開速度,浪費流量以及服務器存儲空間,所以需要在后端處理完圖片再上傳,這里我們用到了Thumbnails圖片處理工具類。
Thumbnails主要支持以下一些功能
1、指定大小進行縮放
2、按照比例進行縮放
3、不按照比例,指定大小進行縮放
4、旋轉
5、水印
6、裁剪
7、轉化圖片格式
8、輸出到OutputStream
9、輸出到BufferedImage
使用步驟:
一、添加Maven
<dependency> <groupId>net.coobird</groupId> <artifactId>thumbnailator</artifactId> <version>0.4.8</version></dependency>
二、具體操作
1:指定大小進行縮放
/** * 指定大小進行縮放 * * @throws IOException */ private void test1() throws IOException { /* * size(width,height) 若圖片橫比200小,高比300小,不變 * 若圖片橫比200小,高比300大,高縮小到300,圖片比例不變 若圖片橫比200大,高比300小,橫縮小到200,圖片比例不變 * 若圖片橫比200大,高比300大,圖片按比例縮小,橫為200或高為300 */ Thumbnails.of('images/test.jpg').size(200, 300).toFile('C:/image_200x300.jpg'); Thumbnails.of('images/test.jpg').size(2560, 2048).toFile('C:/image_2560x2048.jpg'); }
2:按照比例進行縮放
/** * 按照比例進行縮放 * scale 圖片的壓縮比例 值在0-1之間,1f就是原圖,0.5就是原圖的一半大小 * outputQuality 圖片壓縮的質量 值在0-1 之間,越接近1質量越好,越接近0 質量越差 * @throws IOException */ private void test2() throws IOException { /** * scale(比例) */ Thumbnails.of('images/test.jpg').scale(0.25f).outputQuality(0.8f).toFile('C:/image_25%.jpg'); Thumbnails.of('images/test.jpg').scale(0.75f).outputQuality(0.8f).toFile('C:/image_110%.jpg'); }
3:不按照比例,指定大小進行縮放
/** * 不按照比例,指定大小進行縮放 * * @throws IOException */ private void test3() throws IOException { /** * keepAspectRatio(false) 默認是按照比例縮放的 */ Thumbnails.of('images/test.jpg').size(120, 120).keepAspectRatio(false).toFile('C:/image_120x120.jpg'); }
4:旋轉
/** * 旋轉 * * @throws IOException */ private void test4() throws IOException { /** * rotate(角度),正數:順時針 負數:逆時針 */ Thumbnails.of('images/test.jpg').size(1280, 1024).rotate(90).toFile('C:/image+90.jpg'); Thumbnails.of('images/test.jpg').size(1280, 1024).rotate(-90).toFile('C:/iamge-90.jpg'); }
5:水印
/** * 水印 * * @throws IOException */ private void test5() throws IOException { /** * watermark(位置,水印圖,透明度) */ Thumbnails.of('images/test.jpg').size(1280, 1024).watermark(Positions.BOTTOM_RIGHT, ImageIO.read(new File('images/watermark.png')), 0.5f).outputQuality(0.8f).toFile('C:/image_watermark_bottom_right.jpg'); Thumbnails.of('images/test.jpg').size(1280, 1024).watermark(Positions.CENTER, ImageIO.read(new File('images/watermark.png')), 0.5f).outputQuality(0.8f).toFile('C:/image_watermark_center.jpg'); }
6:裁剪
/** * 裁剪 * * @throws IOException */ private void test6() throws IOException { /** * 圖片中心400*400的區域 */ Thumbnails.of('images/test.jpg').sourceRegion(Positions.CENTER, 400, 400).size(200, 200).keepAspectRatio(false).toFile('C:/image_region_center.jpg'); /** * 圖片右下400*400的區域 */ Thumbnails.of('images/test.jpg').sourceRegion(Positions.BOTTOM_RIGHT, 400, 400).size(200, 200).keepAspectRatio(false).toFile('C:/image_region_bootom_right.jpg'); /** * 指定坐標 */ Thumbnails.of('images/test.jpg').sourceRegion(600, 500, 400, 400).size(200, 200).keepAspectRatio(false).toFile('C:/image_region_coord.jpg'); }
7:轉化圖片格式
/** * 轉化圖片格式 * * @throws IOException */ private void test7() throws IOException { /** * outputFormat(圖像格式) */ Thumbnails.of('images/test.jpg').size(1280, 1024).outputFormat('png').toFile('C:/image_1280x1024.png'); Thumbnails.of('images/test.jpg').size(1280, 1024).outputFormat('gif').toFile('C:/image_1280x1024.gif'); }
8:輸出到OutputStream
/** * 輸出到OutputStream * * @throws IOException */ private void test8() throws IOException { /** * toOutputStream(流對象) */ OutputStream os = new FileOutputStream('C:/image_1280x1024_OutputStream.png'); Thumbnails.of('images/test.jpg').size(1280, 1024).toOutputStream(os); }
9:輸出到BufferedImage
/** * 輸出到BufferedImage * * @throws IOException */ private void test9() throws IOException { /** * asBufferedImage() 返回BufferedImage */ BufferedImage thumbnail = Thumbnails.of('images/test.jpg').size(1280, 1024).asBufferedImage(); ImageIO.write(thumbnail, 'jpg', new File('C:/image_1280x1024_BufferedImage.jpg')); }
三、對圖片文件進行Base64操作
/** * 對內存中的圖片文件進行Base64處理 * * @throws IOException */ public String Base64ImageByMemory(BufferedImage pic) { String imgString = ''; ByteArrayOutputStream newBaos = new ByteArrayOutputStream();//io流 try { ImageIO.write(pic, 'jpg', newBaos);//寫入流中 byte[] bytes = newBaos.toByteArray();//轉換成字節 imgString = URLEncoder.encode(new BASE64Encoder().encode(bytes), 'UTF-8'); } catch (Exception e) { e.printStackTrace(); } return imgString; }
四、獲取服務器圖片文件大小
/** * 輸出到OutputStream * * @throws IOException */ public void getImageFileSize(){ int size; URLConnection conn; try { String path=''; path='https://bkimg.cdn.bcebos.com/pic/a8773912b31bb051c36044e93b7adab44bede0af';//世界地圖 //path='http://10.30.23.217:9017/image/0c09ca36-abea-4efa-85b0-99b6d261f66c'; //服務器上圖片 URL url = new URL(path); conn = url.openConnection(); size = conn.getContentLength(); if (size < 0){ System.out.println('Could not determine file size.'); }else{ System.out.println('The size of file is = ' + size + ' bytes'); BigDecimal filesize = new BigDecimal(size); BigDecimal megabyte = new BigDecimal(1024 * 1024); float returnValue = filesize.divide(megabyte, 2, BigDecimal.ROUND_UP).floatValue(); System.out.println('The size of file is = '+returnValue+'M'); } conn.getInputStream().close(); } catch (IOException e) { e.printStackTrace(); } }
至此,圖片壓縮的相關處理和Base64以及獲取服務器文件大小的功能就總結完了!
以上就是Java 使用Thumbnails對大圖片壓縮的詳細內容,更多關于java 大圖片壓縮的資料請關注好吧啦網其它相關文章!
相關文章:
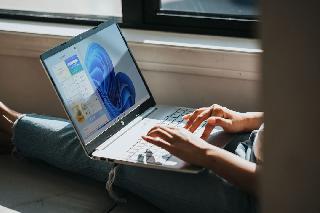